27. March 2006
Bob
IIS , Scripting
Recently I had to work with a customer that was trying to use a 3rd-party utility that read W3C log files and it was failing to complete processing. I had the customer send me his log files, and upon examination I discovered that the trouble was occuring because the customer had been experimenting with adding and removing the different fields from their log files and this was causing the log parsing utility to crash.
As luck would have it, IIS provides a useful logging utility object that you can read more about at the following URL:
I had used this logging utility object for an earlier project, so I was familiar with how it worked. With that knowledge in mind, I wrote the following script that loops through all of the log files in a folder and creates new log files in a subfolder that contain only the default W3C fields. (BTW - I sent this script to the customer and he was able to parse all of his log files successfully. ;-] )
Option Explicit
Randomize Timer
' Declare variables.
Dim objIISLog
Dim objFSO, objFolder, objFile
Dim objOutputFile, strInputFile
Dim strOutputFile, strOutputPath
Dim strLogRecord
Dim blnExists
' Create file system object.
Set objFSO = WScript.CreateObject("Scripting.FileSystemObject")
' Retrieve an object For the current folder.
Set objFolder = objFSO.GetFolder(".")
' Create a subfolder with a random name.
blnExists = True
Do While blnExists = True
strOutputPath = objFolder.Path & "\" & CreateRandomName(20)
blnExists = objFSO.FolderExists(strOutputPath)
Loop
objFSO.CreateFolder strOutputPath
' Loop through the log files in the current folder.
For Each objFile In objFolder.Files
' Test for a log file.
If Right(LCase(objFile.Name),4) = ".log" Then
' Format the file names/paths.
strInputFile = objFolder.Path & "\" & objFile.Name
strOutputFile = strOutputPath & "\" & objFile.Name
' Create and open an IIS logging object.
Set objIISLog = CreateObject("MSWC.IISLog")
' Open the input log file.
objIISLog.OpenLogFile strInputFile, 1, "", 0, ""
' Open the output log file.
Set objOutputFile = objFSO.CreateTextFile(strOutputFile)
' Read the initial record from the log file.
objIISLog.ReadLogRecord
' Write the headers to the output log file.
objOutputFile.WriteLine "#Software: Microsoft Internet Information Services 5.0"
objOutputFile.WriteLine "#Version: 1.0"
objOutputFile.WriteLine "#Date: " & BuildDateTime(objIISLog.DateTime)
objOutputFile.WriteLine "#Fields: date time c-ip cs-username s-ip s-port " & _
"cs-method cs-uri-stem cs-uri-query sc-status cs(User-Agent)"
' Loop through the records in the log file.
Do While Not objIISLog.AtEndOfLog
' Format the log file fields.
strLogRecord = BuildDateTime(objIISLog.DateTime)
strLogRecord = strLogRecord & _
" " & FormatField(objIISLog.ClientIP) & _
" " & FormatField(objIISLog.UserName) & _
" " & FormatField(objIISLog.ServerIP) & _
" " & FormatField(objIISLog.ServerPort) & _
" " & FormatField(objIISLog.Method) & _
" " & FormatField(objIISLog.URIStem) & _
" " & FormatField(objIISLog.URIQuery) & _
" " & FormatField(objIISLog.ProtocolStatus) & _
" " & FormatField(objIISLog.UserAgent)
' Write the output log file record.
objOutputFile.WriteLine strLogRecord
' Read the next record from the log file.
objIISLog.ReadLogRecord
Loop
' Close the input log file.
objIISLog.CloseLogFiles 1
objIISLog = Null
End If
Next
' Inform the user that the operation has completed.
MsgBox "Finished!"
' Format a log file field.
Function FormatField(tmpField)
On Error Resume Next
FormatField = "-"
If Len(tmpField) > 0 Then FormatField = Trim(tmpField)
End Function
' Format a log file date.
Function BuildDateTime(tmpDateTime)
On Error Resume Next
tmpDateTime = CDate(tmpDateTime)
BuildDateTime = Year(tmpDateTime) & "-" & _
Right("0" & Month(tmpDateTime),2) & "-" & _
Right("0" & Day(tmpDateTime),2) & " " & _
Right("0" & Hour(tmpDateTime),2) & ":" & _
Right("0" & Minute(tmpDateTime),2) & ":" & _
Right("0" & Second(tmpDateTime),2)
End Function
' Create a random name.
Function CreateRandomName(intNameLength)
Const strValidChars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
Dim tmpX, tmpY, tmpZ
For tmpX = 1 To intNameLength
tmpY = Mid(strValidChars,Int(Rnd(1)*Len(strValidChars))+1,1)
tmpZ = tmpZ & tmpY
Next
CreateRandomName = tmpZ
End Function
Happy coding!
Note: This blog was originally posted at http://blogs.msdn.com/robert_mcmurray/
20. September 2001
Bob
IIS , SSL , Windows
When you manage a certificate server, you will periodically need to issue certificates to requestors. To to so, use the following steps:
- Open the "Certificate Authority" administrative tool:
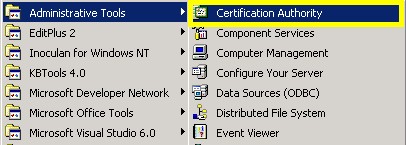
- Click on "Pending Requests":
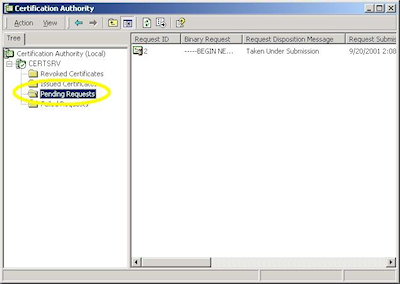
- Right-click the pending request and choose "All Tasks", then click "Issue":
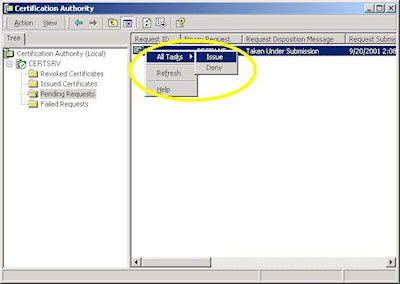
- The certificate will now show up under "Issued Certificates":
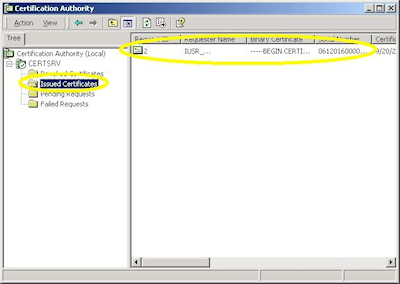
20. September 2001
Bob
IIS , SSL , Windows
Obtaining a root certificate is one of the most important steps for servers or clients that will use certificates that you issue. While this step is not necessary on the server where you installed Certificate Services, it is absolutely essential on your other servers or clients because it allows those computers to trust you as a Certificate Authority. Without that trust in place, you will either receive error messages or SSL simply won't work.
This process is broken into two steps:
- Browse to your certificate server's address, (e.g. http://<server-name>/certsrv/), and choose to retrieve the CA certificate:
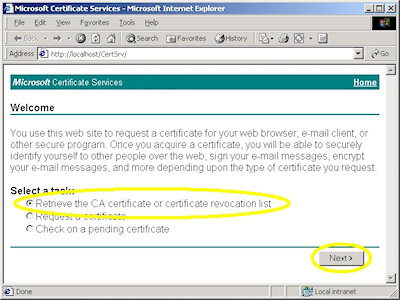
- Click the link to download the CA certificate:
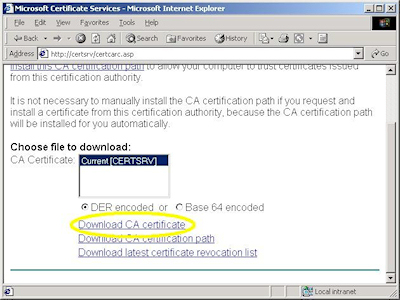
- Choose to save the certificate file to disk:
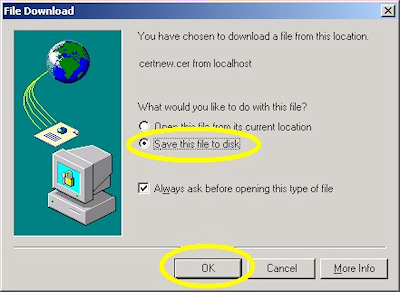
- Save the file to your desktop:
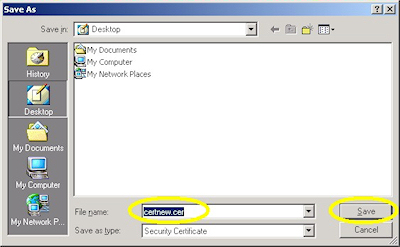
Before using any certificates that you issue on a computer, you need to install the Root Certificate. (This includes web servers and clients.)
- Double-click the file on your desktop:
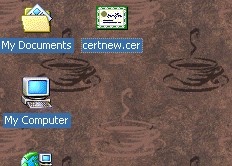
- Click the "Install Certificate" button:
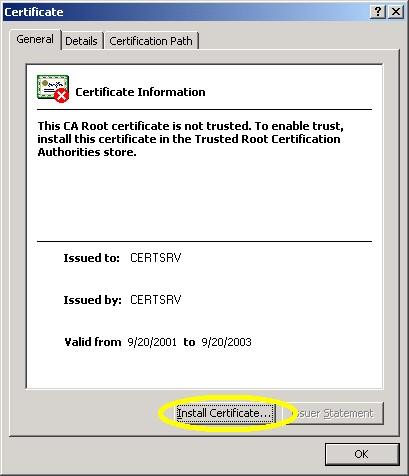
- Click "Next" to start the Certificate Import Wizard:
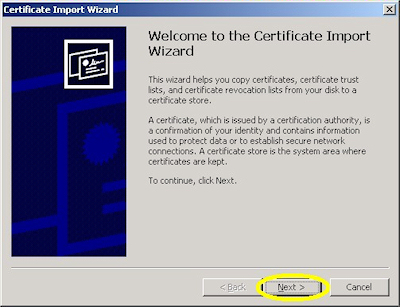
- Choose to automatically choose the store:
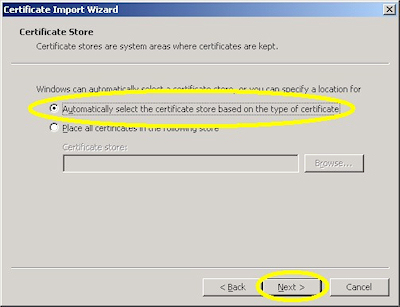
- Click the "Finish" button:
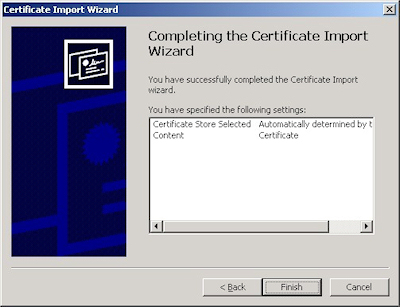
- Click "Yes" when asked if you want to add the certificate:
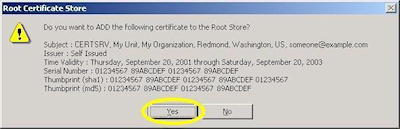
NOTE: This step is very important. If you do not see this dialog, something went wrong.
- Click "OK" when informed that the import was successful.
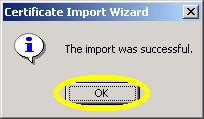
20. September 2001
Bob
IIS , SSL , Windows
In this blog post I'll discuss installing Certificate Services for Windows 2000 in order to test SSL in your environment. To install Certificate Services, use the following steps:
- Run the "Windows Component Wizard" in "Add/Remove Programs", choose "Certificate Services", and click "Next":
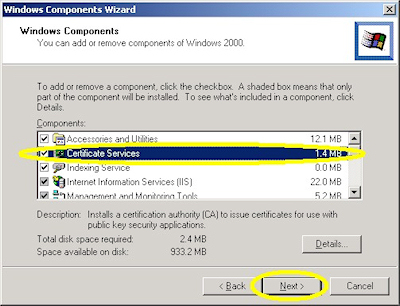
- Choose "Stand-alone root CA", then click "Next":
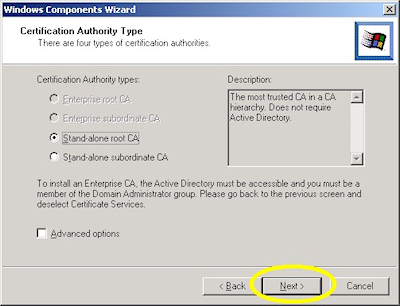
- Enter all requested information, then click "Next":
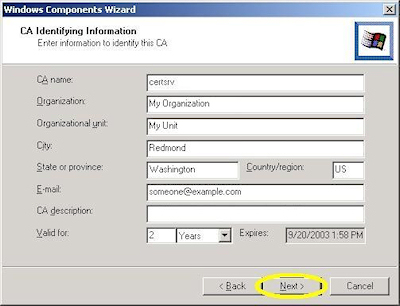
- Accept the defaults for the data locations and click "Next":
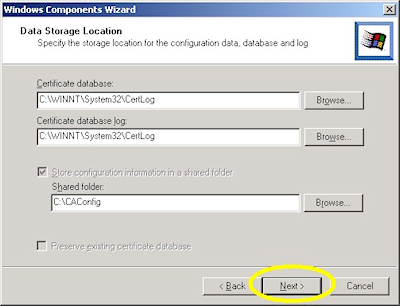
- The wizard will step through installing the services:
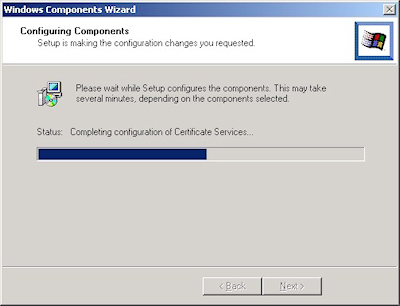
- When the wizard has completed, click "Finish" to exit the wizard:
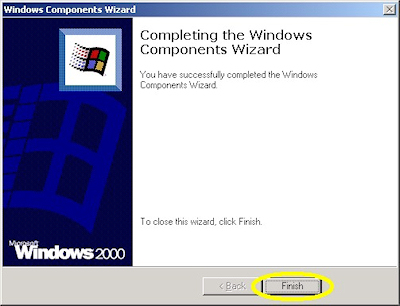
20. September 2001
Bob
IIS , SSL
Typically you would submit your certificate request to any one of several Certificate Authorities (CA). There are several that are available, but here are just a few:
The steps to obtain a certificate differ for each CA, and it would be way outside the scope of my limited blogspace to include the steps for every CA on the Internet. So for my blog series I'm going to show how to use Certificate Services on Windows 2000 to obtain a certificate. This process is broken into three steps:
- Submit the Certificate Request
- Certificate Processing
- Obtain the Certificate
- Browse to the "Certificate Services" web site, choose to "Request a Certificate", then click "Next":
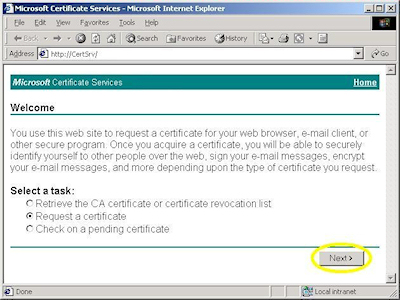
- Choose "Advanced request", then click "Next":
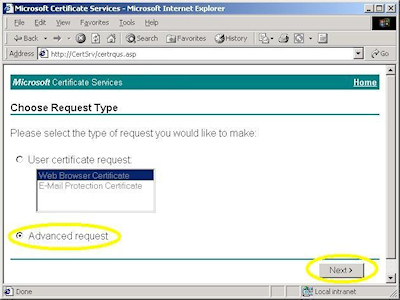
- Choose the option to submit a request using a base64 encoded file, then click "Next":
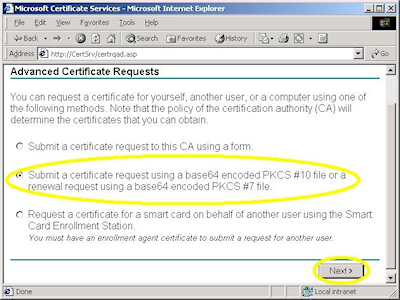
- Copy the text from your certificate request file and paste it into the "Base64 Encoded Certificate Request" text box, then click "Submit":
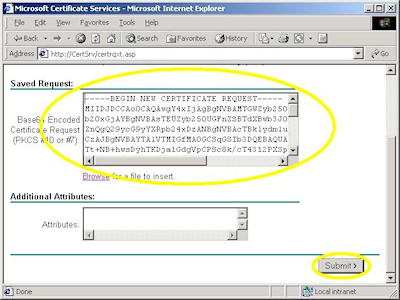
- By default, Certificate Services will return a message stating that your certificate is pending. You will need to notify your Certificate Services administrator that your certificate needs to be approved.
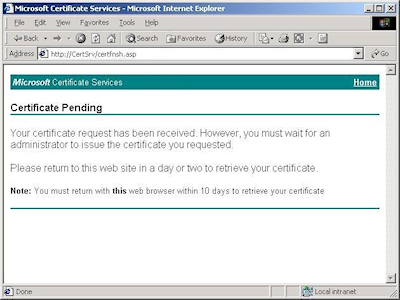
At this point the Certificate Authority (CA) will consider your request. (See processing a Certificate Request for details on issuing your own requests.)
- Browse to the "Certificate Services" web site, choose to "Check on a Pending Certificate", then click "Next":
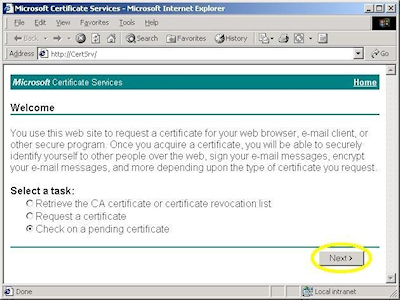
- Highlight your approved request, then click "Next":
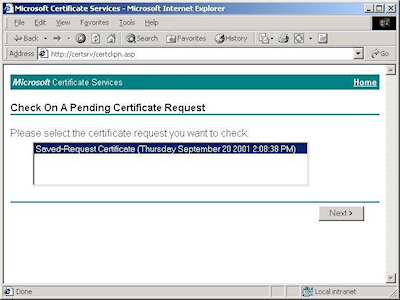
- Click the link to "Download CA certificate":
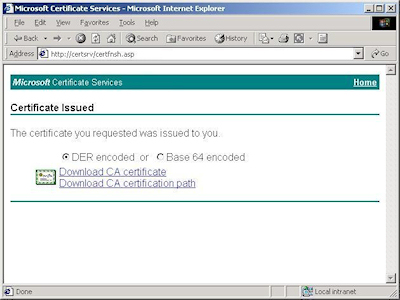
- When prompted, choose to save the file to disk, then click "OK":
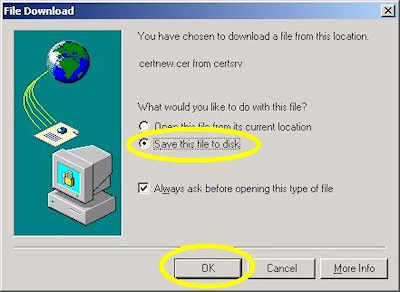
- Save the file to somewhere convenient, like your desktop:
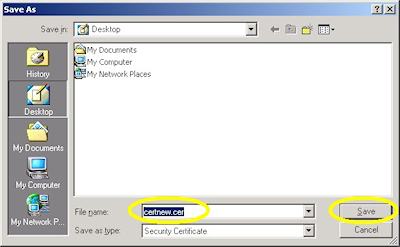
- When you have saved the file to your computer, Windows Explorer will display the file with an icon that indicates that it contains a certificate:
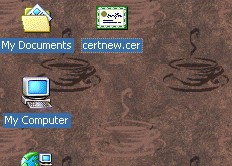
20. September 2001
Bob
IIS , SSL
- Bring up the properties for a web site:
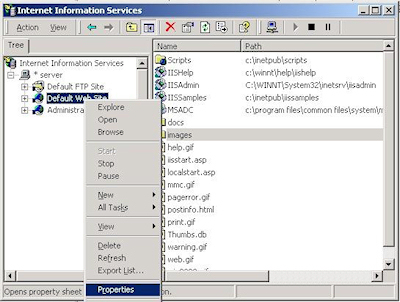
- Switch to the "Directory Security" tab and click "Server Certificate:"
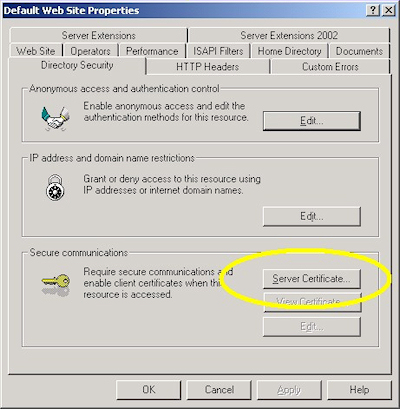
- Click "Next" to bypass the first page:
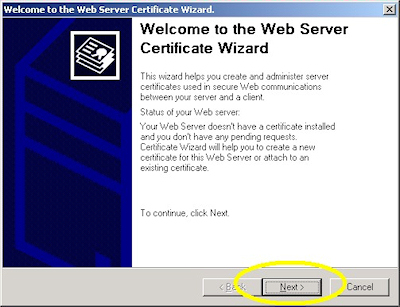
- Choose to "Create a new certificate" and click "Next":
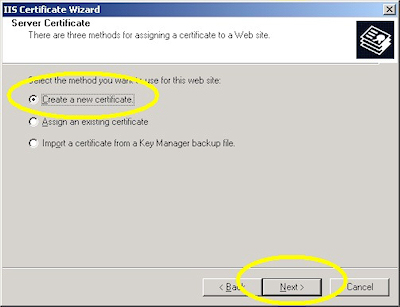
- Choose to "Prepare the request now, but send later" and click "Next":
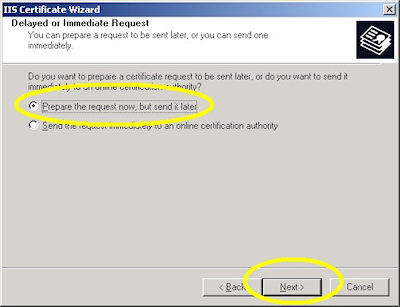
- Enter a friendly "Name" for the request, and your desired "Bit length". (SGC certificates are only necessary for overseas customers.) Click "Next":
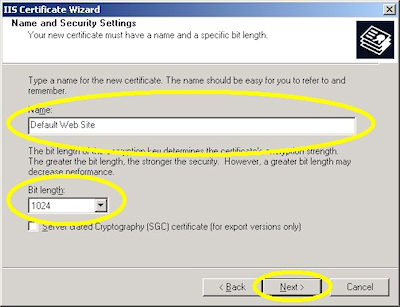
- Enter your "Organization" and "Organization unit", then click "Next":
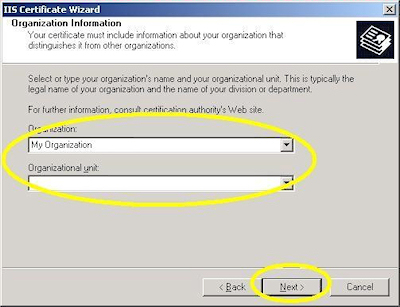
- Enter the "Common name" for your site then click "Next":
Note: This must be the actual web address that users will browse to when they hit your site.
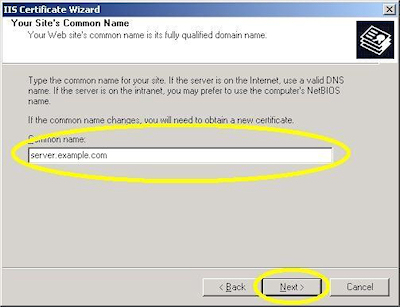
- Enter your "Country", "State", and "City", then click "Next":
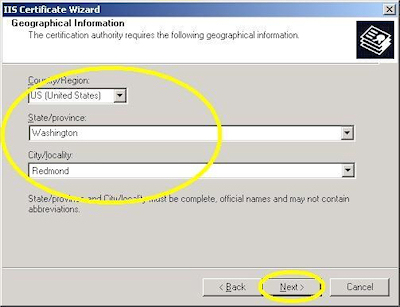
- Enter the "File name" for your request, then click Next:
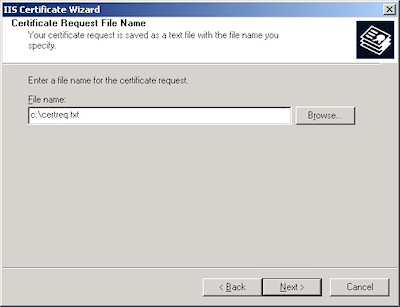
- Review the information for your request, then click Next:
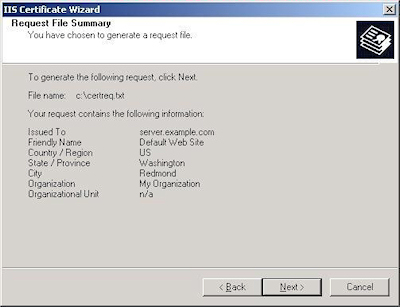
- Click "Finish" to exit the wizard.
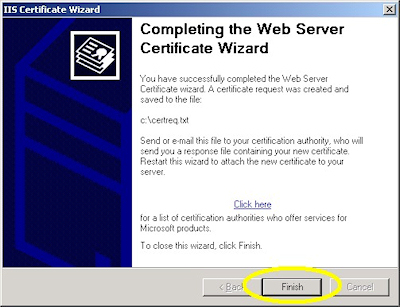
FYI: If you were to open your request file in Notepad, it will look something like the following:
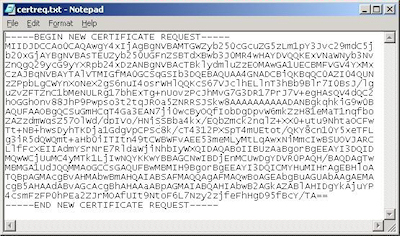